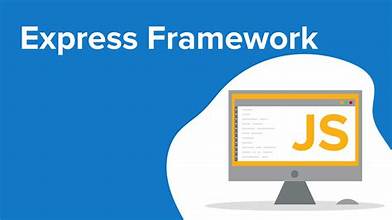
Express.js, commonly referred to as Express, is a flexible and minimalist web application framework for Node.js, a popular runtime environment for executing JavaScript code outside of a web browser. Express simplifies the process of building web applications and APIs by providing a robust set of features and utilities, allowing developers to create scalable and efficient server-side applications with ease.
Table of Contents:
- Introduction to Express.js
- History and Evolution
- Architecture of Express
- Key Features of Express
- Express Middleware
- Routing in Express
- Handling Requests and Responses
- Template Engines and Views
- Express and RESTful APIs
- Error Handling in Express
- Working with Databases
- Authentication and Authorization
- Security Best Practices
- Performance Optimization
- Testing Express Applications
- Deployment Strategies
- Express Ecosystem
- Case Studies and Examples
- Future of Express.js
1. Introduction to Express.js:
Express.js is a lightweight and unopinionated web framework designed for building web applications and APIs with Node.js. It provides a minimalistic and flexible structure, allowing developers to create server-side applications using JavaScript, the same language used for client-side programming in web browsers.
2. History and Evolution:
Express.js was created by TJ Holowaychuk and initially released in 2010. Since then, it has gained widespread adoption and has become one of the most popular web frameworks for Node.js. Express has evolved over the years with regular updates and contributions from the open-source community.
3. Architecture of Express:
Express follows a middleware-oriented architecture, where incoming HTTP requests are passed through a series of middleware functions before being routed to the appropriate handler. This modular architecture promotes code reusability, separation of concerns, and extensibility.
4. Key Features of Express:
- Robust routing system
- Middleware support for processing requests
- Integration with template engines for dynamic content generation
- Built-in support for handling HTTP requests and responses
- Simplified error handling mechanism
- Support for RESTful API development
- Extensibility through third-party middleware and plugins
5. Express Middleware:
Middleware functions in Express are functions that have access to the request object (req
), the response object (res
), and the next middleware function in the application’s request-response cycle. Middleware functions can perform tasks such as parsing request bodies, logging, authentication, and error handling.
6. Routing in Express:
Express provides a powerful routing system that allows developers to define routes for handling different HTTP methods (GET, POST, PUT, DELETE, etc.) and URL patterns. Routes are defined using a simple and intuitive syntax, making it easy to create complex routing configurations.
7. Handling Requests and Responses:
Express simplifies the process of handling HTTP requests and generating responses by providing methods and utilities for working with request and response objects. Developers can easily read request headers, parameters, and bodies, and send back appropriate responses with status codes and data.
8. Template Engines and Views:
Express integrates with various template engines such as Pug (formerly known as Jade), EJS, and Handlebars for generating dynamic HTML content on the server-side. Template engines allow developers to create reusable layouts and partials, making it easier to maintain and manage views.
9. Express and RESTful APIs:
Express is well-suited for building RESTful APIs due to its lightweight and modular architecture. Developers can define routes for handling CRUD (Create, Read, Update, Delete) operations on resources and implement common API features such as pagination, sorting, and filtering.
10. Error Handling in Express:
Express provides a built-in error handling mechanism that allows developers to define error-handling middleware functions to catch and process errors that occur during request processing. Error-handling middleware functions can be used to log errors, send error responses, or perform other custom error handling logic.
11. Working with Databases:
Express can be used with a variety of databases including relational databases like MySQL, PostgreSQL, and SQLite, as well as NoSQL databases like MongoDB and Redis. Developers can use database drivers and ORMs (Object-Relational Mappers) to interact with databases and perform data manipulation operations.
12. Authentication and Authorization:
Express provides support for implementing authentication and authorization mechanisms to secure web applications and APIs. Developers can use middleware functions and third-party packages to handle user authentication, manage user sessions, and enforce access control policies.
13. Security Best Practices:
Security is a crucial aspect of web application development, and Express offers features and best practices for improving the security posture of applications. This includes techniques for preventing common security vulnerabilities such as cross-site scripting (XSS), SQL injection, and CSRF (Cross-Site Request Forgery) attacks.
14. Performance Optimization:
Express applications can be optimized for performance by implementing techniques such as caching, compression, and asynchronous processing. Developers can leverage tools and strategies for profiling and tuning application performance to ensure optimal responsiveness and scalability.
15. Testing Express Applications:
Testing is an essential part of software development, and Express provides tools and libraries for writing unit tests, integration tests, and end-to-end tests for applications. Developers can use testing frameworks like Mocha, Chai, and Supertest to automate the testing process and ensure the reliability of their applications.
16. Deployment Strategies:
Express applications can be deployed using various deployment strategies including traditional web hosting, containerization with Docker, and serverless computing with platforms like AWS Lambda and Azure Functions. Developers can choose the deployment strategy that best fits the requirements of their application.
17. Express Ecosystem:
The Express ecosystem includes a wide range of third-party middleware, plugins, and extensions that extend the capabilities of the framework. These include middleware for authentication, session management, logging, and more, as well as plugins for integrating with databases, caching systems, and other services.
18. Case Studies and Examples:
Many companies and organizations have successfully built and deployed web applications and APIs using Express.js. Case studies and examples showcase the versatility and scalability of Express for various use cases and industries.
19. Future of Express.js:
The future of Express.js looks promising, with continued support and updates from the open-source community. As Node.js and JavaScript continue to gain popularity for web development, Express.js is expected to remain a popular choice for building server-side applications and APIs.
This comprehensive guide provides a detailed overview of Express.js, covering its features, architecture, best practices, and ecosystem. Whether you’re a beginner learning to use Express.js or an experienced developer looking to deepen your knowledge, this guide serves as a valuable resource for understanding and mastering the Express.js framework.