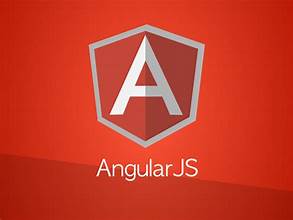
AngularJS is not a programming language per se; it’s a JavaScript framework maintained by Google. However, it’s often referred to as a language due to its unique syntax and the way it structures code. Let’s delve into what AngularJS is all about:
Title: Exploring AngularJS: The JavaScript Framework
Introduction
AngularJS is a powerful front-end JavaScript framework developed and maintained by Google. It’s designed to simplify the development and testing of dynamic web applications by providing a structured framework for building client-side applications. AngularJS extends HTML with new attributes and binds data to HTML with expressions, making it a popular choice for building Single Page Applications (SPAs).
Table of Contents
- Understanding AngularJS
- 1.1 What is AngularJS?
- 1.2 Historical Context
- 1.3 Key Features
- Core Concepts of AngularJS
- 2.1 Data Binding
- 2.2 Controllers
- 2.3 Directives
- 2.4 Services
- 2.5 Modules
- Programming in AngularJS
- 3.1 Setting Up an AngularJS Project
- 3.2 Building Components with AngularJS
- 3.3 Routing and Navigation
- 3.4 Forms and Validation
- 3.5 Working with HTTP Requests
- Advanced Topics in AngularJS
- 4.1 Dependency Injection
- 4.2 Testing AngularJS Applications
- 4.3 Internationalization and Localization
- 4.4 AngularJS Best Practices
- 4.5 Performance Optimization
- Practical Applications
- 5.1 Single Page Applications (SPAs)
- 5.2 Web Development Projects
- 5.3 Enterprise Applications
- 5.4 Mobile Applications
- 5.5 Real-time Applications
- Tools and Resources
- 6.1 Angular CLI
- 6.2 Developer Tools
- 6.3 Community Support
- 6.4 Documentation and Tutorials
- 6.5 Online Courses and Training
- Challenges and Limitations
- 7.1 Learning Curve
- 7.2 Performance Overhead
- 7.3 Compatibility Issues
- 7.4 Version Migration
- Future Trends
- 8.1 AngularJS vs. Angular
- 8.2 Adoption in the Industry
- 8.3 Integration with Other Technologies
- 8.4 Evolution of the Framework
- Conclusion
1. Understanding AngularJS
1.1 What is AngularJS?
AngularJS is an open-source JavaScript framework developed by Google for building dynamic web applications. It extends HTML syntax to express the components of an application clearly and succinctly. AngularJS follows the Model-View-Controller (MVC) architectural pattern and provides a rich set of features for building interactive and data-driven web applications.
1.2 Historical Context
AngularJS was first released by Google in 2010, with the goal of simplifying web development and promoting best practices for building client-side applications. It gained rapid adoption due to its powerful features and developer-friendly approach. Over time, AngularJS evolved, with multiple versions released to address performance, scalability, and usability concerns.
1.3 Key Features
AngularJS is known for its key features, including:
- Data Binding: Automatic synchronization of data between the model and view components.
- Directives: Extending HTML with custom attributes and behaviors.
- Dependency Injection: Managing dependencies and promoting modularity in code.
- Routing: Supporting navigation and routing between different views in a single-page application.
- Testing: Built-in support for unit testing and end-to-end testing of AngularJS applications.
2. Core Concepts of AngularJS
2.1 Data Binding
Data binding in AngularJS allows synchronization of data between the model and the view automatically. Changes in the model are reflected in the view, and vice versa, without the need for manual intervention.
2.2 Controllers
Controllers in AngularJS are JavaScript functions that are responsible for handling business logic and interacting with the view and model components. They provide a way to organize and manage application logic.
2.3 Directives
Directives are markers on a DOM element that tell AngularJS’s HTML compiler ($compile
) to attach a specified behavior to that DOM element or even transform the DOM element and its children.
2.4 Services
Services in AngularJS are reusable components that provide functionality across an application. They are often used for tasks such as data manipulation, HTTP requests, and business logic.
2.5 Modules
Modules in AngularJS provide a way to organize an application into reusable components. They encapsulate related controllers, services, directives, filters, and configuration information.
3. Programming in AngularJS
3.1 Setting Up an AngularJS Project
Setting up an AngularJS project involves including the AngularJS library, defining modules, and configuring routing. Tools like Angular CLI can streamline the project setup process.
3.2 Building Components with AngularJS
Components are the building blocks of AngularJS applications. They encapsulate HTML, CSS, and JavaScript functionality into reusable and self-contained units.
3.3 Routing and Navigation
AngularJS’s built-in routing module allows developers to define navigation paths and load different views based on URL changes. This enables the creation of single-page applications with multiple views.
3.4 Forms and Validation
AngularJS provides built-in support for form handling and validation. Developers can use directives and services to create dynamic forms with client-side validation logic.
3.5 Working with HTTP Requests
AngularJS includes an HTTP module for making HTTP requests to remote servers. Developers can use this module to fetch data from APIs and interact with backend services.
4. Advanced Topics in AngularJS
4.1 Dependency Injection
Dependency injection in AngularJS promotes modularity and testability by allowing components to declare their dependencies explicitly. AngularJS’s injector subsystem handles the instantiation and management of dependencies.
4.2 Testing AngularJS Applications
AngularJS provides built-in support for testing applications using tools like Jasmine and Karma. Developers can write unit tests and end-to-end tests to ensure the reliability and correctness of their AngularJS applications.
4.3 Internationalization and Localization
AngularJS supports internationalization (i18n) and localization (l10n) out of the box. Developers can use AngularJS’s localization features to create multilingual applications that adapt to different languages and locales.
4.4 AngularJS Best Practices
Following best practices is essential for building maintainable and scalable AngularJS applications. Best practices include organizing code structure, optimizing performance, handling error and exception, and implementing security measures.
4.5 Performance Optimization
AngularJS applications can benefit from performance optimization techniques such as lazy loading, code splitting, tree shaking, and caching. These techniques help improve the loading time and responsiveness of AngularJS applications, especially in large-scale projects.
5. Practical Applications
5.1 Single Page Applications (SPAs)
AngularJS is well-suited for building single-page applications (SPAs) that provide a seamless and interactive user experience. SPAs load once and dynamically update content as users navigate
the application.
5.2 Web Development Projects
AngularJS is widely used in web development projects of various sizes and complexities. It powers numerous websites, web applications, and enterprise solutions across different industries.
5.3 Enterprise Applications
AngularJS’s robust features, scalability, and modularity make it an ideal choice for building enterprise-grade applications. It’s used in customer relationship management (CRM), enterprise resource planning (ERP), and other business-critical systems.
5.4 Mobile Applications
AngularJS can be used for developing cross-platform mobile applications using frameworks like Ionic and NativeScript. These frameworks leverage AngularJS’s capabilities to build native-like mobile applications for iOS and Android platforms.
5.5 Real-time Applications
AngularJS can be integrated with real-time technologies like WebSockets and Server-Sent Events (SSE) to build real-time applications such as chat applications, live dashboards, and collaborative tools.
6. Tools and Resources
6.1 Angular CLI
Angular CLI is a command-line interface tool that helps developers scaffold, build, and maintain AngularJS applications. It provides commands for generating components, services, modules, and other AngularJS artifacts.
6.2 Developer Tools
Developer tools like AngularJS Batarang, Augury, and ng-inspector provide debugging and profiling capabilities for AngularJS applications. These tools help developers analyze performance, inspect application state, and debug issues during development.
6.3 Community Support
The AngularJS community is active and vibrant, with forums, mailing lists, and social media channels where developers can seek help, share knowledge, and collaborate on projects.
6.4 Documentation and Tutorials
AngularJS’s official documentation is comprehensive and well-maintained, providing guidance on various aspects of AngularJS development. Additionally, there are numerous tutorials, blog posts, and online courses available for learning AngularJS.
6.5 Online Courses and Training
Online platforms like Udemy, Coursera, and Pluralsight offer a wide range of courses and training programs for learning AngularJS. These courses cover beginner to advanced topics and provide hands-on exercises and projects for practical learning.
7. Challenges and Limitations
7.1 Learning Curve
AngularJS has a steep learning curve, especially for developers new to front-end development or JavaScript frameworks. Understanding core concepts like data binding, dependency injection, and routing requires time and practice.
7.2 Performance Overhead
AngularJS applications may suffer from performance overhead, especially in large-scale projects with complex views and heavy data binding. Careful optimization and performance tuning are necessary to mitigate these issues.
7.3 Compatibility Issues
AngularJS’s rapid evolution and version updates may lead to compatibility issues between different versions of AngularJS or third-party libraries. Version management and compatibility testing are essential for maintaining stability in AngularJS projects.
7.4 Version Migration
Migrating from older versions of AngularJS to newer versions or from AngularJS to Angular (formerly known as Angular 2+) can be challenging and time-consuming. It requires updating code, resolving breaking changes, and ensuring compatibility with new features and APIs.
8. Future Trends
8.1 AngularJS vs. Angular
The release of Angular (Angular 2+) introduced significant changes and improvements over AngularJS. Angular offers better performance, improved modularity, and enhanced tooling compared to AngularJS. However, AngularJS continues to be used in existing projects and legacy systems.
8.2 Adoption in the Industry
AngularJS remains a popular choice for web development projects, especially in enterprises and large organizations. While newer frameworks like React and Vue.js have gained traction, AngularJS continues to have a significant presence in the industry.
8.3 Integration with Other Technologies
AngularJS can be integrated with other technologies and frameworks to extend its capabilities and address specific requirements. Integration with server-side technologies, databases, authentication providers, and third-party APIs enhances AngularJS’s versatility and applicability.
8.4 Evolution of the Framework
The AngularJS framework continues to evolve, with updates and enhancements released regularly to address performance, security, and usability concerns. Future versions of AngularJS may focus on improving developer experience, optimizing performance, and supporting emerging web standards.
9. Conclusion
AngularJS is a powerful JavaScript framework for building dynamic and interactive web applications. It provides developers with a structured framework, rich set of features, and comprehensive tooling for developing modern web applications. While AngularJS has its challenges and limitations, its popularity, versatility, and vibrant ecosystem make it a compelling choice for web development projects of all sizes and complexities.
This comprehensive overview aims to provide insights into AngularJS, covering its key features, core concepts, programming techniques, advanced topics, practical applications, challenges, future trends, and resources. Whether you’re a beginner exploring front-end development or an experienced developer seeking to enhance your skills, AngularJS offers a robust platform for building modern web applications.