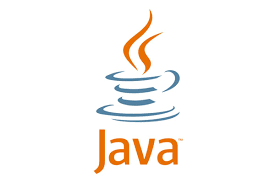
Sure, here are 10 important Java questions and answers that cover various aspects of the language:
- What is Java?
Java is a high-level, object-oriented programming language developed by Sun Microsystems (now owned by Oracle). It is known for its platform independence, which means Java programs can run on any device that has the Java Virtual Machine (JVM) installed. - What are the main features of Java?
- Object-oriented
- Platform independence
- Robust and secure
- Multi-threaded
- Portable
- Dynamic
- What is the difference between JDK, JRE, and JVM?
- JDK (Java Development Kit): It includes the tools needed to develop Java applications, such as the compiler and debugger.
- JRE (Java Runtime Environment): It contains the JVM and other libraries needed to run Java applications.
- JVM (Java Virtual Machine): It is the runtime environment in which Java bytecode is executed.
- What is the difference between an interface and an abstract class in Java?
- An interface can only contain abstract methods and constants, while an abstract class can contain both abstract and concrete methods.
- A class can implement multiple interfaces but can only extend one abstract class.
- What is the difference between == and .equals() in Java?
- == is used to compare the reference or memory address of objects.
- .equals() is a method used to compare the contents or values of objects.
- What is the difference between static and non-static methods in Java?
- Static methods belong to the class and can be called without creating an instance of the class.
- Non-static methods belong to the object and can only be called on an instance of the class.
- What is the purpose of the ‘final’ keyword in Java?
- The ‘final’ keyword is used to make a variable, method, or class immutable or unchangeable.
- When applied to a variable, it indicates that its value cannot be modified once initialized.
- When applied to a method, it prevents the method from being overridden in subclasses.
- When applied to a class, it prevents the class from being subclassed.
- What is the difference between StringBuffer and StringBuilder?
- StringBuffer is synchronized and thread-safe, while StringBuilder is not synchronized and not thread-safe.
- StringBuilder is generally faster than StringBuffer, but StringBuffer is preferred in multithreaded environments due to its thread safety.
- What is exception handling in Java?
Exception handling in Java is a mechanism to handle runtime errors or exceptional situations that occur during the execution of a program. It involves the use of try, catch, and finally blocks to handle exceptions gracefully. - What is the purpose of the ‘static’ keyword in Java?
- The ‘static’ keyword is used to declare variables, methods, and nested classes that belong to the class rather than instances of the class.
- Static variables are shared among all instances of a class.
- Static methods can be called without creating an instance of the class.
- Static nested classes are associated with the outer class and can be instantiated without an instance of the outer class.