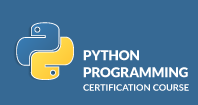
list of 50 Python questions along with multiple-choice answer options:
- What is Python?
- A) A high-level programming language
- B) A type of snake
- C) An operating system
- D) A database management system
- Answer: A) A high-level programming language
- Which of the following is not a Python data type?
- A) Integer
- B) List
- C) String
- D) Float Point
- Answer: D) Float Point
- What is the output of
print(2 * 3 ** 3 % 5)
?
- A) 2
- B) 3
- C) 4
- D) 0
- Answer: C) 4
- What is the output of
print("Hello" + 3 * "World")
?
- A) HelloWorld
- B) Hello3World
- C) HelloWorldWorldWorld
- D) HelloHelloHelloWorld
- Answer: C) HelloWorldWorldWorld
- What does the
len()
function do in Python?
- A) Returns the length of a string
- B) Returns the length of a list
- C) Returns the length of an object
- D) All of the above
- Answer: D) All of the above
- Which of the following is the correct way to comment multiple lines in Python?
- A) // This is a comment
- B) # This is a comment
- C) /* This is a comment */
- D) ”’ This is a comment ”’
- Answer: D) ”’ This is a comment ”’
- What is the output of
print("Python"[::-1])
?
- A) Python
- B) nohtyP
- C) P
- D) TypeError
- Answer: B) nohtyP
- What is the purpose of the
range()
function in Python?
- A) Generates a list of numbers
- B) Generates an arithmetic progression of numbers
- C) Returns a range object
- D) All of the above
- Answer: D) All of the above
- Which of the following statements is true about Python?
- A) Python is a compiled language
- B) Python is a dynamically typed language
- C) Python is not suitable for web development
- D) Python is a low-level language
- Answer: B) Python is a dynamically typed language
- What is the output of
print(10 // 3)
?- A) 3.333
- B) 3
- C) 4
- D) 3.0
- Answer: B) 3
- What is the output of
print(5 == 5.0)
?- A) True
- B) False
- C) Error
- D) None
- Answer: A) True
- Which of the following is a mutable data type in Python?
- A) Tuple
- B) String
- C) List
- D) Set
- Answer: C) List
- What does the
strip()
function do in Python?- A) Removes leading and trailing whitespace
- B) Removes leading whitespace
- C) Removes trailing whitespace
- D) None of the above
- Answer: A) Removes leading and trailing whitespace
- What is the output of
print("hello".upper())
?- A) hello
- B) HELLO
- C) Hello
- D) Error
- Answer: B) HELLO
- What is the correct way to define a function in Python?
- A) function my_function():
- B) define my_function():
- C) def my_function():
- D) func my_function():
- Answer: C) def my_function():
- What is the output of
print(10 > 9 and 5 < 4)
?- A) True
- B) False
- C) Error
- D) None
- Answer: B) False
- Which of the following is not a valid Python data structure?
- A) Stack
- B) Queue
- C) Heap
- D) Table
- Answer: D) Table
- What is the output of
print("python".capitalize())
?- A) python
- B) Python
- C) PYTHON
- D) Error
- Answer: B) Python
- What does the
append()
method do in Python?- A) Adds an element to the end of a list
- B) Adds an element to the beginning of a list
- C) Adds an element to a tuple
- D) Adds an element to a dictionary
- Answer: A) Adds an element to the end of a list
- What is the output of
print(2 ** 3 ** 2)
?- A) 512
- B) 64
- C) 72
- D) 9
- Answer: A) 512
- What is the purpose of the
zip()
function in Python?- A) Combines two lists into a list of tuples
- B) Unzips a list of tuples into separate lists
- C) Creates a compressed file
- D) None of the above
- Answer: A) Combines two lists into a list of tuples
- What is the output of
print("Python"[0])
?- A) P
- B) y
- C) h
- D) Error
- Answer: A) P
- Which of the following is not a valid way to open a file in Python?
- A) open(“file.txt”, “r”)
- B) open(“file.txt”, “w”)
- C) open(“file.txt”, “a”)
- D) open(“file.txt”, “execute”)
- Answer: D) open(“file.txt”, “execute”)
- What is the output of
print("Hello, World!".split(", "))
?- A) [‘Hello’, ‘World!’]
- B) [‘Hello,’, ‘World!’]
- C) (‘Hello’, ‘World!’)
- D) Error
- Answer: A) [‘Hello’, ‘World!’]
- What does the
sorted()
function do in Python?- A) Sorts a list in ascending order
- B) Sorts a list in descending order
- C) Sorts a dictionary by keys
- D) All of the above
- Answer: A) Sorts a list in ascending order
- What is the output of
print("Hello" * 3)
?- A)
- B) Hello 3 times
- C) HelloHello
- D) Error
- Answer: A) HelloHelloHello
- Which of the following is the correct way to check if a key exists in a dictionary in Python?
- A) if key in dict:
- B) if dict.has_key(key):
- C) if dict[key]:
- D) if dict.exists(key):
- Answer: A) if key in dict:
- What is the output of
print("Python"[1:4])
?- A) P
- B) y
- C) yth
- D) ytho
- Answer: C) yth
- What does the
pop()
method do in Python?- A) Removes and returns the last element of a list
- B) Removes and returns the first element of a list
- C) Removes and returns an element from a specific index of a list
- D) Removes and returns an element from a specific key of a dictionary
- Answer: C) Removes and returns an element from a specific index of a list
- What is the output of
print(not(5 > 3))
?- A) True
- B) False
- C) Error
- D) None
- Answer: B) False
- Which of the following is a correct way to define a tuple in Python?
- A) (1, 2, 3)
- B) {1, 2, 3}
- C) [1, 2, 3]
- D) “1, 2, 3”
- Answer: A) (1, 2, 3)
- What is the output of
print(10 / 3)
?- A) 3.333
- B) 3
- C) 4
- D) 3.0
- Answer: A) 3.333
- What does the
isdigit()
method do in Python?- A) Returns True if all characters in a string are digits
- B) Returns True if all characters in a string are alphabetic
- C) Returns True if all characters in a string are alphanumeric
- D) Returns True if all characters in a string are lowercase
- Answer: A) Returns True if all characters in a string are digits
- What is the output of
print(10 != 5)
?- A) True
- B) False
- C) Error
- D) None
- Answer: A) True
- Which of the following is the correct way to define a set in Python?
- A) {1, 2, 3}
- B) [1, 2, 3]
- C) (1, 2, 3)
- D) “1, 2, 3”
- Answer: A) {1, 2, 3}
- What is the output of
print(2 + 2 * 3)
?- A) 8
- B) 10
- C) 6
- D) Error
- Answer: C) 6
- What does the
join()
method do in Python?- A) Joins elements of a list into a string
- B) Joins elements of a string into a list
- C) Joins elements of a tuple into a list
- D) None of the above
- Answer: A) Joins elements of a list into a string
- What is the output of
print("Python".lower())
?- A) Python
- B) python
- C) PYTHON
- D) Error
- Answer: B) python
- Which of the following is not a valid way to declare a variable in Python?
- A) var_name = value
- B) varName = value
- C) VarName = value
- D) 1var = value
- Answer: D) 1var = value
- What is the output of
print("Python"[::-1])
?- A) Python
- B) nohtyP
- C) P
- D) TypeError
- Answer: B) nohtyP
- What is the purpose of the
map()
function in Python?- A) Applies a function to each item in an iterable
- B) Creates a map object
- C) Filters elements from an iterable
- D) None of the above
- Answer: A) Applies a function to each item in an iterable
- What is the output of
print("Hello".find("l"))
?- A) 0
- B) 2
- C) 3
- D) Error
- Answer: B) 2
- Which of the following is a correct way to define a dictionary in Python?
- A) {1: ‘one’, 2: ‘two’, 3: ‘three’}
- B) [1: ‘one’, 2: ‘two’, 3: ‘three’]
- C) (1: ‘one’, 2: ‘two’, 3: ‘three’)
- D) “1: ‘one’, 2: ‘two’, 3: ‘three'”
- Answer: A) {1: ‘one’, 2: ‘two’, 3: ‘three’}
- What is the output of
print(10 % 3)
?- A) 3.333
- B) 3
- C) 1
- D) 0
- Answer: C) 1
- What does the
extend()
method do in Python?- A) Adds elements from one list to another list
- B) Adds an element to the end of a list
- C) Adds an element to the beginning of a list
- D) Adds an element to a tuple
- Answer: A) Adds elements from one list to another list
- What is the output of
print(10 < 5 or 5 > 3)
?- A) True
- B) False
- C) Error
- D) None
- Answer: A) True
- What is the output of
print("Hello"[-1])
?- A) H
- B) e
- C) o
- D) Error
- Answer: C) o
- Which of the following is not a valid way to create a tuple in Python?
- A) (1, 2, 3)
- B) tuple(1, 2, 3)
- C) (1, 2, 3,)
- D) (1, 2,
- Answer: B) tuple(1, 2, 3)
- What is the output of
print("Python"[1:3])
?- A) P
- B) y
- C) yt
- D) th
- Answer: C) yt
- What is the output of
print("Python"[2:])
?- A) Py
- B) y
- C) thon
- D) None
- Answer: C) thon