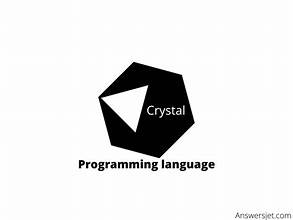
Crystal Programming Language: A Comprehensive Guide
Introduction to Crystal:
Crystal is a modern, statically typed, and compiled programming language designed for simplicity, performance, and developer productivity. It combines the elegance of Ruby’s syntax with the speed and efficiency of a compiled language like C. Crystal is well-suited for building web applications, command-line tools, and system utilities.
History of Crystal:
Origins:
- The development of Crystal began in 2011 by Ary Borenszweig and Juan Wajnerman as a personal project.
- Crystal was inspired by Ruby and aimed to bring its expressive syntax to a statically typed language with compile-time type checking.
Release:
- Crystal’s first commit was made in 2011, and it was open-sourced in 2014 under the MIT license.
- The language gained traction quickly due to its familiar syntax for Ruby developers and its promise of performance improvements.
Community Growth:
- Crystal’s community has grown steadily since its release, with contributors from around the world contributing libraries, tools, and documentation.
- Crystal’s popularity continues to rise as more developers discover its benefits for writing fast and maintainable code.
Features of Crystal:
Statically Typed:
- Crystal is a statically typed language, meaning that variable types are known at compile-time, allowing for early detection of type-related errors.
- Static typing improves code reliability and performance by enabling compiler optimizations.
Ruby-like Syntax:
- Crystal’s syntax is heavily influenced by Ruby, making it easy for Ruby developers to transition to Crystal.
- Crystal features familiar constructs such as classes, modules, methods, blocks, and iterators, making it intuitive and expressive to write.
Compiled:
- Crystal code is compiled ahead-of-time to native machine code, resulting in fast and efficient executables.
- The Crystal compiler leverages LLVM (Low-Level Virtual Machine) for code generation, optimizing performance across different platforms.
Metaprogramming:
- Crystal supports metaprogramming features similar to Ruby, allowing for dynamic code generation and manipulation at compile-time.
- Metaprogramming enables writing concise and expressive code, reducing boilerplate and improving productivity.
Concurrency:
- Crystal provides built-in support for concurrency through lightweight fibers and channels.
- Fibers are lightweight cooperative threads that allow for non-blocking asynchronous execution, while channels facilitate communication and synchronization between concurrent tasks.
Crystal Syntax:
Hello World Example:
puts "Hello, Crystal!"
Variables and Data Types:
- Crystal supports various data types including integers, floats, booleans, characters, strings, arrays, hashes, and user-defined types.
- Variables in Crystal can be declared using the var keyword or with explicit type annotations.
Control Flow:
- Crystal supports traditional control flow constructs such as if statements, loops, switch statements, and exception handling.
- Crystal also features powerful iterators and pattern matching for writing concise and expressive code.
Functions and Methods:
- Functions in Crystal are defined using the def keyword, with optional parameter types and return types.
- Crystal supports both regular functions and methods defined within classes and modules.
Classes and Modules:
- Crystal is an object-oriented language with support for classes, inheritance, mixins, and interfaces.
- Classes in Crystal encapsulate data and behavior, while modules provide namespaces and code organization.
Crystal for Web Development:
Web Frameworks:
- Crystal can be used for web development using frameworks such as Kemal, Lucky, and Amber.
- Kemal is a fast, minimalist web framework inspired by Ruby’s Sinatra, while Lucky provides a full-stack framework with built-in ORM and authentication.
Database Access:
- Crystal provides libraries for interacting with databases such as PostgreSQL, MySQL, SQLite, and MongoDB.
- ORMs (Object-Relational Mappers) like Granite and Avram simplify database access and data modeling in Crystal applications.
Templating Engines:
- Crystal supports templating engines such as Kilt and Crinja for generating dynamic HTML content in web applications.
- Templating engines enable separating logic from presentation, improving code maintainability and readability.
Crystal for System Programming:
Command-Line Tools:
- Crystal is well-suited for building command-line tools and utilities due to its performance and expressive syntax.
- Libraries like Commander and OptionParser facilitate parsing command-line arguments and building CLI applications.
System Libraries:
- Crystal provides bindings to system libraries such as libc and libevent for low-level system programming tasks.
- These libraries enable interacting with the operating system, filesystem, networking, and other system-level operations.
Interoperability:
- Crystal supports interoperability with C code through its C bindings feature, allowing for seamless integration with existing C libraries.
- Interoperability with C code enables leveraging existing libraries and tools in Crystal applications.
Crystal Ecosystem:
Package Manager:
- Crystal’s package manager, called shards, provides a centralized repository for sharing and distributing Crystal libraries.
- Shards simplifies dependency management and makes it easy to integrate third-party libraries into Crystal projects.
Development Tools:
- Crystal developers have access to a range of development tools including editors, IDEs, linters, formatters, and debuggers.
- Editors like Visual Studio Code and Atom provide syntax highlighting and code completion for Crystal, while tools like Crystal Tools enhance the development workflow.
Crystal Community:
Forums and Communities:
- Crystal has a vibrant and welcoming community of developers who share their knowledge, ideas, and experiences through forums, mailing lists, and social media channels.
- Communities like the Crystal Forum, Crystal Chat, and Crystal Meetups provide platforms for discussion, collaboration, and networking.
Conferences and Events:
- Crystal developers organize and participate in conferences, meetups, and hackathons around the world to promote the language and share insights and best practices.
- Events like CrystalConf and Crystal Meetups bring together developers from diverse backgrounds to learn, collaborate, and celebrate their love for Crystal.
Conclusion:
Crystal is a powerful and elegant programming language that combines the expressiveness of Ruby with the performance of a compiled language. Its statically typed nature, Ruby-like syntax, and robust ecosystem make it an attractive choice for building a wide range of applications, from web services and APIs to system utilities and command-line tools. With its growing community and ecosystem, Crystal is poised to become a prominent player in the world of programming languages, empowering developers to write fast, reliable, and maintainable code.
This comprehensive guide provides a detailed overview of the Crystal programming language, covering its history, features, syntax, applications, ecosystem, community, and more. Whether you’re new to Crystal or looking to deepen your understanding, this guide serves as a valuable resource for developers at all levels.