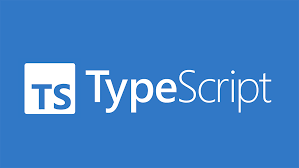
TypeScript is a statically typed superset of JavaScript that adds optional static typing to the language. It is designed for large-scale JavaScript application development, offering enhanced tooling, code organization, and error detection capabilities. Here’s a comprehensive guide to TypeScript, covering its history, usage, features, and more:
History of TypeScript:
TypeScript was developed by Anders Hejlsberg, the creator of C#, and his team at Microsoft. It was first announced in October 2012 and released as an open-source project in October 2012. The primary motivation behind TypeScript’s creation was to address the challenges of developing large-scale JavaScript applications, such as lack of static typing, tooling limitations, and difficulty in maintaining codebases.
Since its release, TypeScript has gained significant adoption and popularity in the JavaScript community, thanks to its powerful features and seamless integration with existing JavaScript codebases. It is actively maintained by Microsoft and has a vibrant ecosystem supported by a growing community of developers and contributors.
Key Features of TypeScript:
- Static Typing: TypeScript introduces static typing to JavaScript, allowing developers to define types for variables, parameters, functions, and more. This helps catch type-related errors at compile time, improving code quality and maintainability.
- Type Inference: TypeScript’s type inference system automatically deduces types based on context, reducing the need for explicit type annotations and making code more concise and readable.
- Interfaces and Classes: TypeScript supports interfaces and classes, enabling developers to define contracts and create object-oriented structures with inheritance, encapsulation, and polymorphism.
- Enums: TypeScript provides support for enums, allowing developers to define named constants with a set of predefined values, making code more expressive and self-documenting.
- Generics: TypeScript supports generics, enabling developers to write reusable and type-safe code components that work with different data types.
- Union Types and Intersection Types: TypeScript allows developers to create union types and intersection types, providing flexibility in defining complex data structures and function signatures.
- Type Guards and Type Assertions: TypeScript offers type guards and type assertions for runtime type checking and casting, improving type safety and error handling in code.
- Decorators: TypeScript supports decorators, which are a form of metadata annotations applied to classes, methods, properties, or parameters. Decorators enable developers to add custom behavior or metadata to their code, such as logging, validation, or dependency injection.
Usage of TypeScript:
- Web Development: TypeScript is widely used for web development, particularly in large-scale projects where strong typing and tooling support are crucial for maintaining code quality and productivity. It is commonly used with popular frontend frameworks like Angular, React, and Vue.js.
- Node.js Development: TypeScript is also popular in the Node.js ecosystem, where it provides enhanced tooling, type safety, and code organization for server-side JavaScript development. Many Node.js frameworks and libraries, such as Express.js and NestJS, offer TypeScript support out of the box.
- Desktop and Mobile Development: TypeScript can be used for building desktop and mobile applications using frameworks like Electron and React Native. Its static typing and tooling support make it well-suited for developing cross-platform applications with JavaScript.
- Backend Development: TypeScript is increasingly being adopted for backend development, thanks to its strong typing, object-oriented features, and compatibility with popular backend frameworks and libraries like NestJS and TypeORM.
- Tooling and Automation: TypeScript is commonly used in build tools, task runners, and automation scripts for tasks such as code linting, transpilation, testing, and deployment. Its static typing and modular architecture make it a powerful tool for enhancing developer workflows and productivity.
Getting Started with TypeScript:
To start using TypeScript in your projects, you’ll need to follow these steps:
- Installation: Install TypeScript globally or locally in your project using npm or yarn:
npm install -g typescript
or
npm install typescript --save-dev
- Setting Up a TypeScript Configuration: Create a
tsconfig.json
file in your project’s root directory to configure TypeScript options:
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"strict": true,
"esModuleInterop": true
},
"include": ["src/**/*.ts"],
"exclude": ["node_modules"]
}
- Writing TypeScript Code: Write your JavaScript code using TypeScript syntax, including type annotations, interfaces, classes, and other TypeScript features:
// Example TypeScript code
function greet(name: string) {
return `Hello, ${name}!`;
}
console.log(greet("World"));
- Compiling TypeScript Code: Use the TypeScript compiler (
tsc
) to compile your TypeScript code into JavaScript:
tsc
This will generate JavaScript files based on your TypeScript code, which you can then run in a browser, Node.js environment, or other JavaScript runtime.
- Integrating with Build Tools: Integrate TypeScript into your build process using tools like webpack, Parcel, or gulp for tasks such as bundling, optimization, and code transformation.
- Testing and Debugging: Test and debug your TypeScript code using tools like Jest, Mocha, Chai, or debuggers integrated with your development environment (e.g., Visual Studio Code, WebStorm).
- Deployment: Deploy your compiled JavaScript code along with any necessary assets (HTML, CSS, etc.) to your hosting environment or server for production use.
Advanced Topics and Best Practices:
- Advanced TypeScript Features: Explore advanced TypeScript features such as async/await, decorators, generics, mapped types, conditional types, and more to enhance your development workflow and codebase.
- Error Handling and Debugging: Learn techniques for error handling, debugging, and troubleshooting TypeScript code using tools like TypeScript’s built-in compiler diagnostics, debugging tools, and error monitoring services.
- Performance Optimization: Optimize the performance of your TypeScript code by following best practices such as minimizing runtime overhead, reducing bundle size, optimizing algorithms and data structures, and leveraging language features for performance improvements.
- Code Organization and Architecture: Adopt best practices for organizing and structuring your TypeScript codebase, including modularization, separation of concerns, design patterns, and architectural principles such as SOLID and DRY.
- Testing and Quality Assurance: Implement testing strategies such as unit testing, integration testing, and end-to-end testing to ensure the correctness, reliability, and robustness of your TypeScript code. Use testing frameworks, libraries, and tools to automate testing processes and validate code behavior.
Conclusion:
TypeScript is a powerful and versatile language that offers static typing, modern features, and enhanced tooling for JavaScript development. Whether you’re building web applications, server-side applications, desktop applications, or mobile apps, TypeScript can help you write safer, more maintainable, and scalable code. By understanding its key features, usage scenarios, and best practices, you can leverage TypeScript to build high-quality software solutions that meet the demands of today’s complex and dynamic development environments.