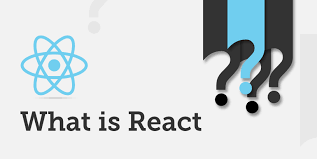
Title: Understanding React.js: A Comprehensive Guide
Introduction:
React.js, commonly referred to as React, is a popular open-source JavaScript library for building user interfaces (UIs) and single-page applications (SPAs). Developed and maintained by Facebook, React has gained widespread adoption due to its declarative and component-based approach, efficient rendering capabilities, and robust ecosystem. This comprehensive guide aims to provide a thorough understanding of React.js, covering its core concepts, features, practical applications, best practices, and future trends.
I. Fundamentals of React.js:
- Introduction to React.js:
- React.js is a JavaScript library for building interactive user interfaces, focusing on component reusability and efficient rendering.
- It was first released by Facebook in 2013 and has since gained significant popularity among developers worldwide.
- Declarative Programming Model:
- React employs a declarative programming paradigm, where developers specify the desired UI state, and React handles the underlying updates.
- This approach simplifies UI development by abstracting away the manual DOM manipulation and state management.
- Component-Based Architecture:
- React encourages the creation of modular, reusable components that encapsulate UI elements and logic.
- Components can be composed hierarchically to build complex UIs, promoting code organization and maintainability.
- Virtual DOM (Document Object Model):
- React utilizes a virtual DOM abstraction to efficiently update the UI in response to changes.
- Instead of directly manipulating the browser’s DOM, React reconciles changes against a virtual representation before applying minimal updates to the actual DOM.
- JSX (JavaScript XML):
- JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript files.
- JSX facilitates the creation of React components by providing a familiar and expressive syntax for defining UI elements.
II. Core Concepts and Features:
- Components:
- Components are the building blocks of React applications, representing reusable UI elements with encapsulated logic.
- React components can be either functional components (stateless) or class components (stateful), depending on their need for internal state management.
- Props (Properties) and State:
- Props are immutable data passed from parent to child components, allowing for dynamic configuration and customization.
- State represents the internal data of a component that can change over time, triggering re-renders and UI updates.
- Lifecycle Methods:
- React components have lifecycle methods that enable developers to hook into specific stages of a component’s lifecycle.
- Lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount facilitate initialization, updates, and cleanup operations.
- Hooks:
- Introduced in React 16.8, hooks are functions that enable functional components to use state and other React features without writing a class.
- useState, useEffect, useContext, and useCallback are popular hooks used for managing state, performing side effects, accessing context, and optimizing performance.
- Context API:
- The Context API provides a mechanism for passing data through the component tree without explicitly passing props at every level.
- Context allows for global state management and facilitates the sharing of data between components that are not directly related.
III. Practical Applications:
- Building User Interfaces:
- React.js is widely used for building dynamic and interactive user interfaces, ranging from simple web pages to complex SPAs.
- Its component-based architecture and virtual DOM reconciliation make it suitable for designing responsive and scalable UIs.
- Single-Page Applications (SPAs):
- React is particularly well-suited for developing SPAs, where the entire application is loaded once, and subsequent interactions are handled dynamically.
- Frameworks like React Router enable client-side routing and navigation within SPAs, enhancing the user experience.
- Mobile App Development:
- React Native, a framework based on React.js, allows developers to build cross-platform mobile applications using JavaScript and React.
- With React Native, developers can leverage a single codebase to target multiple platforms like iOS and Android, reducing development time and effort.
- Progressive Web Applications (PWAs):
- React.js can be used to develop PWAs, which combine the features of web and native mobile applications for enhanced performance and offline capabilities.
- Service workers, caching strategies, and manifest files enable PWAs built with React to deliver a native-like experience to users.
- Integrations and Ecosystem:
- React.js integrates seamlessly with other libraries, frameworks, and tools, such as Redux for state management, GraphQL for data fetching, and Webpack for bundling assets.
- The React ecosystem boasts a vast array of third-party libraries, components, and utilities, catering to diverse development needs and use cases.
IV. Best Practices and Optimization Techniques:
- Component Reusability:
- Emphasize component reusability by designing small, composable components that serve a single responsibility.
- Use props for configuring components and passing data between parent and child components.
- State Management:
- Adopt a centralized state management approach, especially for complex applications with multiple interconnected components.
- Consider using libraries like Redux or the useContext hook for managing global or shared state.
- Performance Optimization:
- Optimize performance by minimizing unnecessary re-renders and rendering only the components affected by state changes.
- Utilize memoization techniques, pure components, and React.memo to prevent unnecessary renders and improve rendering performance.
- Code Splitting and Lazy Loading:
- Implement code splitting techniques to split large bundles into smaller chunks, improving initial load times and reducing time to interactive.
- Utilize React.lazy and Suspense to lazily load components and resources only when needed, enhancing performance and resource utilization.
- Accessibility and SEO:
- Ensure accessibility by adhering to web content accessibility guidelines (WCAG) and providing keyboard navigation, focus management, and semantic HTML.
- Improve search engine optimization (SEO) by pre-rendering content on the server-side using frameworks like Next.js or Gatsby.js.
V. Future Trends and Advancements:
- Concurrent Mode and Suspense:
- React’s Concurrent Mode aims to improve the responsiveness and interactivity of applications by prioritizing high-priority updates and deferring low-priority updates.
- Suspense introduces a declarative way to handle loading states, enabling components to suspend rendering while waiting for asynchronous data.
- Server Components:
- Server Components, an experimental feature in React, enables components to be rendered on the server and streamed to the client, improving server-side rendering performance and scalability.
- React 18 and Beyond:
- React 18 is expected to introduce new features, optimizations, and APIs focused on performance, concurrency, and developer experience.
- Community-driven initiatives and advancements in the React ecosystem will continue to shape the future of React.js development.
Conclusion:
React.js has emerged as a leading framework for building modern web applications, offering a powerful combination of simplicity, performance, and flexibility. By embracing its component-based architecture, declarative programming model, and ecosystem of tools and libraries, developers can create scalable, maintainable, and user-friendly applications across various platforms and devices. As React.js continues to evolve and innovate, it remains a cornerstone of web development, driving forward the boundaries of user experience and developer productivity.