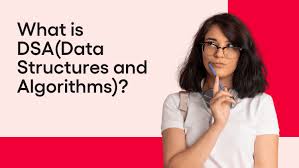
Title: Understanding Data Structures and Algorithms: A Comprehensive Exploration
Introduction:
In the realm of computer science, data structures and algorithms (DSA) form the backbone of efficient problem-solving and software development. This comprehensive guide aims to delve into the intricacies of data structures and algorithms, exploring their history, fundamental concepts, practical applications, and advanced techniques.
I. Fundamentals of Data Structures and Algorithms:
- Definition and Importance:
- Data structures refer to the organization, storage, and manipulation of data in a computer program.
- Algorithms are step-by-step procedures or methods for solving computational problems.
- Understanding DSA is crucial for developing efficient and scalable software solutions.
- Characteristics of Good Data Structures and Algorithms:
- Efficiency: Data structures and algorithms should optimize resource usage, such as time and memory.
- Correctness: Algorithms must produce accurate results for all valid inputs.
- Maintainability: DSA should be easy to understand, modify, and maintain.
- Scalability: Solutions should scale gracefully with increasing input sizes.
II. Historical Overview of Data Structures and Algorithms:
- Early Developments:
- The concept of data structures dates back to ancient civilizations, with early examples like lists and arrays.
- Algorithms have a rich history, with ancient mathematicians developing techniques for arithmetic operations and problem-solving.
- Mathematical Foundations:
- Formalized mathematical concepts laid the groundwork for modern data structures and algorithms.
- Contributions from mathematicians like Carl Friedrich Gauss and Pierre-Simon Laplace influenced computational methods and algorithms.
- Early Computing Era:
- The emergence of electronic computers in the mid-20th century catalyzed advancements in data structures and algorithms.
- Early pioneers like Alan Turing and John von Neumann contributed to theoretical frameworks and algorithmic principles.
- Algorithmic Innovations:
- Landmark algorithms such as Dijkstra’s algorithm for shortest paths and the Knuth–Morris–Pratt algorithm for string searching revolutionized problem-solving techniques.
- The development of efficient sorting algorithms like Quicksort and mergesort paved the way for algorithmic optimization.
- Evolution of Data Structures:
- From simple arrays and linked lists to complex structures like trees, graphs, and hash tables, data structure design evolved to address diverse computational needs.
- The advent of object-oriented programming (OOP) led to the development of abstract data types (ADTs), providing encapsulation and modularity.
III. Core Data Structures:
- Arrays and Lists:
- Arrays are contiguous blocks of memory used to store elements of the same type.
- Linked lists consist of nodes connected by pointers, allowing dynamic memory allocation and efficient insertion/deletion.
- Stacks and Queues:
- Stacks follow the Last In, First Out (LIFO) principle and support operations like push and pop.
- Queues adhere to the First In, First Out (FIFO) principle and support enqueue and dequeue operations.
- Trees and Binary Trees:
- Trees are hierarchical data structures comprising nodes connected by edges, with each node having zero or more children.
- Binary trees are special trees where each node has at most two children, commonly used in search algorithms and expression evaluation.
- Graphs and Graph Algorithms:
- Graphs represent networks of interconnected nodes (vertices) and edges, facilitating modeling of real-world relationships.
- Graph algorithms address various problems like shortest paths, minimum spanning trees, and graph traversal.
- Hash Tables and Hashing:
- Hash tables use a hash function to map keys to indices, enabling efficient key-value pair storage and retrieval.
- Hashing is a fundamental technique employed in data storage, indexing, and cryptography.
IV. Fundamental Algorithms:
- Searching Algorithms:
- Linear search and binary search are fundamental searching techniques used to locate elements within a collection of data.
- Advanced algorithms like depth-first search (DFS) and breadth-first search (BFS) traverse graphs and trees to find specific elements or paths.
- Sorting Algorithms:
- Sorting algorithms rearrange elements in a specified order, such as numerical or lexicographical.
- Classic sorting algorithms include bubble sort, selection sort, insertion sort, mergesort, and quicksort, each with unique efficiency characteristics.
- Greedy Algorithms:
- Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum.
- Examples include Dijkstra’s algorithm for shortest paths and Huffman coding for data compression.
- Dynamic Programming:
- Dynamic programming breaks down complex problems into simpler subproblems and stores solutions to avoid redundant computations.
- Widely used in optimization problems, dynamic programming algorithms exhibit optimal substructure and overlapping subproblems.
V. Advanced Data Structures and Algorithms:
- Advanced Trees and Graphs:
- Red-black trees, AVL trees, and B-trees are balanced tree structures optimized for efficient insertion, deletion, and search operations.
- Advanced graph algorithms tackle complex problems like network flow, connectivity, and matching.
- String Algorithms:
- String manipulation algorithms address tasks such as pattern matching, substring search, and text compression.
- Techniques like finite automata, suffix arrays, and dynamic programming enhance string processing capabilities.
- Geometric Algorithms:
- Geometric algorithms deal with computational geometry problems involving points, lines, polygons, and other geometric entities.
- Applications include collision detection, convex hull computation, and spatial indexing.
- Parallel and Distributed Algorithms:
- Parallel algorithms exploit multiple processing units to accelerate computation and enhance scalability.
- Distributed algorithms coordinate multiple interconnected systems to achieve common goals, vital for distributed systems and network protocols.
VI. Practical Applications:
- Software Engineering:
- DSA concepts underpin the design, implementation, and optimization of software systems across various domains.
- Data structures and algorithms influence software architecture, database design, and algorithmic trading systems.
- Artificial Intelligence and Machine Learning:
- DSA play a crucial role in the development of AI and machine learning algorithms for pattern recognition, predictive modeling, and optimization.
- Data structures like graphs and trees are instrumental in representing knowledge graphs, decision trees, and neural networks.
- Computer Graphics and Gaming:
- Rendering engines and game frameworks utilize data structures and algorithms to efficiently process geometric data, simulate physics, and manage game states.
- Spatial data structures like quad trees and octrees facilitate efficient collision detection and scene management in 3D environments.
- Big Data and Analytics:
- Data structures and algorithms power big data platforms and analytics tools for processing, querying, and analyzing vast datasets.
- Techniques like MapReduce, distributed hash tables, and graph algorithms enable scalable data processing and mining.
VII. Challenges and Future Directions:
- Scalability and Performance:
- As data volumes continue to grow exponentially, scalability and performance remain critical challenges for DSA practitioners.
- Distributed computing, parallel algorithms, and hardware acceleration techniques aim to address scalability concerns.
- Algorithmic Complexity:
- Theoretical analysis of algorithmic complexity and runtime performance helps identify bottlenecks and optimize critical paths.
- Research into approximation algorithms, quantum algorithms, and randomized algorithms explores new avenues for complexity management.
- Privacy and Security:
- The proliferation of data-driven technologies raises concerns about data privacy, security, and algorithmic bias.
- Ethical considerations and regulatory frameworks aim to safeguard user privacy, mitigate security risks, and promote algorithmic fairness.
- Emerging Technologies:
- Advances in quantum computing, bioinformatics, and AI pose new